Navigating the Dynamics 365 ecosystem can be daunting, especially when facing errors like the Dataverse EntityState exception. This comprehensive guide simplifies these complex concepts, offering clear explanations and practical solutions for beginners.
Introduction to EntityState Exceptions in Dynamics 365 / Dataverse
As organizations increasingly adopt Microsoft Dynamics 365 for managing customer relationships and enterprise resources, understanding its backend structure, like Dataverse, becomes crucial. One common challenge users encounter is the EntityState exception. This article breaks down the Dataverse EntityState exception into digestible parts, providing a roadmap for troubleshooting and resolving these errors without the SEO jargon overload.
EntityState must be set to null, Created (for Create message) or Changed (for Update message)
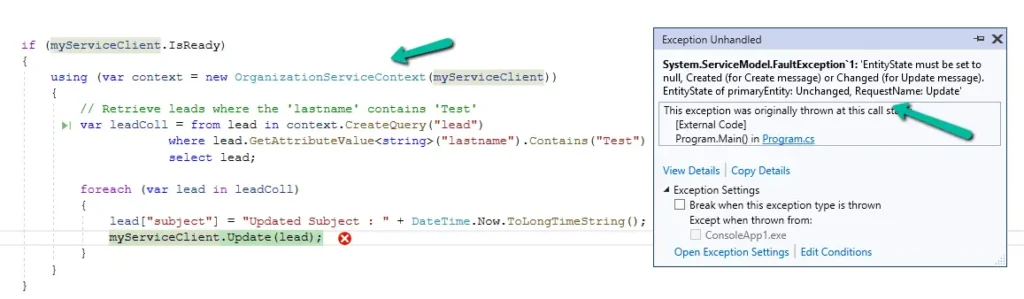
What is EntityState in Dataverse?
EntityState is a critical concept within Dataverse used to define the state of a data entity. It signals whether an entity, such as a record within a database, is new, modified, or unchanged. By managing EntityState effectively, Dynamics 365 can track changes efficiently, ensuring that only necessary updates are pushed through the system.
Understanding the Dynamics 365 EntityState Error
Entity State errors in Dynamics 365 typically arise when there’s a mismatch in how an entity’s state is defined versus how it is supposed to be handled. This discrepancy can cause significant disruptions, leading to unsuccessful create or update operations.
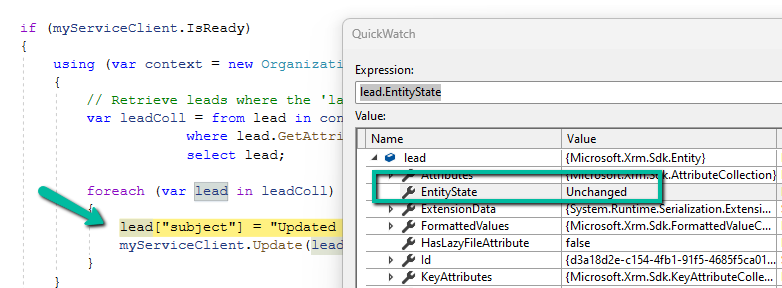
Why is EntityState Set to Null Causing Errors?
When the EntityState is set to null, it becomes ambiguous for Dataverse to determine the intended action for that entity. Typically, this should not be the case since EntityState should clearly denote whether the entity is set for creation, modification, or left unchanged. Null values can trigger exceptions, leading to failed transactions or updates.
Dynamics 365 Record Update Issues
Unintended errors during record updates are quite common in Dynamics 365. These typically occur due to incorrect Entity State settings, where an entity’s state does not match the operation trying to be executed. For instance, a record listed as ‘Unchanged’ should not invoke an update operation.
Table: Common EntityStates and Their Meanings
EntityState | Description |
---|---|
Null | Undefined state, causing errors in operations |
Created | A new entity ready to be added to the database |
Changed | An existing entity that has been modified |
Unchanged | An entity that has not been altered and requires no update |
Dataverse Exception Handling
Exception handling in Dataverse involves implementing structured mechanisms to catch and resolve these EntityState errors. Here’s a step-by-step approach:
Identify the Error: Trace the error back to the exact operation where it occurred. This usually involves reviewing logs or error messages.
Understand the Context: Determine if the issue stems from creation (Entity State: Created), update (Entity State: Changed), or an unknown state (Entity State: Null).
Correct Entity State: Adjust the Entity State to reflect the correct action. For instance, set the Entity State to ‘Created’ for new records or ‘Changed’ for modified ones.
Reattempt the Operation: Once corrected, execute the operation again to determine if the error persists.
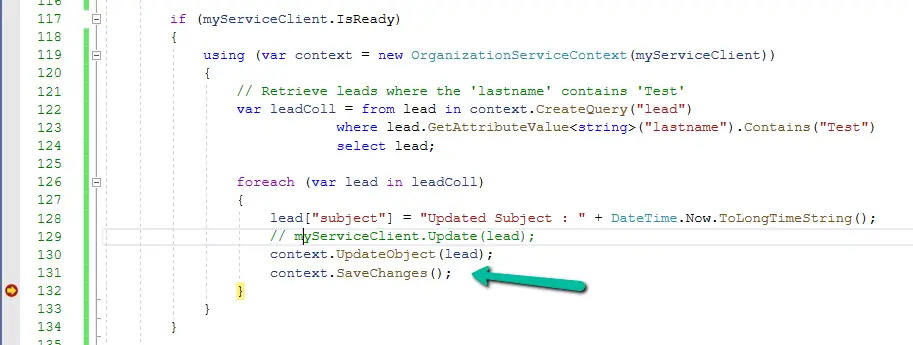
How to Fix EntityState Exception in Dynamics 365
Fixing EntityState exceptions requires methodical troubleshooting:
Step 1: Verify your workflow or plugin logic to ensure it sets the appropriate EntityState.
Step 2: Utilize transaction-level tracing to pinpoint where the exception occurs within the process flow.
Step 3: Check if recent customizations or updates inadvertently altered entity states.
Step 4: Modify and test the logic in a sandbox environment before deploying the corrections to production.
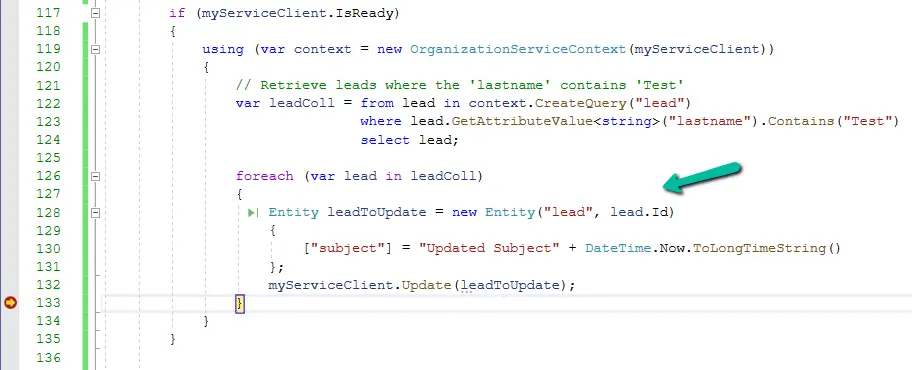
Sample Code
if (myServiceClient.IsReady)
{
using (var context = new OrganizationServiceContext(myServiceClient))
{
// Retrieve leads where the 'lastname' contains 'Test'
var leadColl = from lead in context.CreateQuery("lead")
where lead.GetAttributeValue<string>("lastname").Contains("Test")
select lead;
// use Update Object
foreach (var lead in leadColl)
{
lead.Attributes["subject"] = "Updated Subject" + DateTime.Now.ToLongTimeString();
context.UpdateObject(lead);
context.SaveChanges();
}
// or create a new Entity object
foreach (var lead in leadColl)
{
Entity leadToUpdate = new Entity("lead", lead.Id)
{
["subject"] = "Updated Subject" + DateTime.Now.ToLongTimeString()
};
myServiceClient.Update(leadToUpdate);
}
}
}
Resolving EntityState Exceptions in Dynamics 365 and Resolving Dataverse Update Errors
Dataverse update errors often stem from incorrect Entity State settings. Employing robust validation checks and ensuring the entity’s status aligns with the desired operation are vital steps. Implement logging mechanisms to capture the full context of the operation, which can shed light on potential mismatches leading to failures.
Conclusion
Understanding and handling EntityState exceptions in Dataverse is crucial for streamlined operations within Dynamics 365. By grasping the essence of Entity State and employing structured error-handling approaches, businesses can mitigate disruptions, ensuring efficient data management and operation accuracy. With these insights, managing such errors becomes a more straightforward task, laying the groundwork for a more reliable CRM experience.
Frequently Asked Questions (FAQs)
What is Entity State in Dataverse?
EntityState indicates the current status of an entity (like a record) in Dataverse, informing the system whether it’s new, altered, or unchanged.
How to fix EntityState exception in Dynamics 365?
Identify the error, review the logic setting EntityState, and make sure it aligns with the intended operation. Correct it and retry the transaction.
Why is Entity State set to null causing errors?
Entity State being null leaves its operation undefined, making it unclear if the entity is new or altered, leading to transaction errors.
What does Entity State Unchanged mean?
‘Unchanged’ means the entity has not been modified since last checked, signaling that no updates should be processed.
How to handle update errors in Dataverse?
Ensure Entity State reflects the operation (e.g., ‘Changed’ for updates), utilize logging for error tracking, and validate entity logic.
#MSFTAdvocate #AbhishekDhoriya #LearnWithAbhishekDhoriya #DynamixAcademy
References & Read More:
- Demystifying CMake Script Debugging on Linux with Visual Studio: A Beginner’s Guide
- Unveiling the Future: How Microsoft leads the AI Revolution in Customer Service?
- Mastering Item Reservations in Business Central: A Comprehensive Guide for Beginners
- Mastering Business Central Location Setup: A Comprehensive Guide for Beginners
- Understanding Financial Reports in Business Central: Step by Step Beginner’s Guide
- Microsoft Business Applications Launch Event 2024