Introduction to How to Create Folders in SharePoint Online with PnP Framework and C#?
SharePoint Online is a powerful platform that organizations use for collaboration and document management. However, it can sometimes be daunting for beginners to perform certain tasks, like checking if a folder exists and creating it if it doesn’t. Today, we’ll walk you through an easy, step-by-step guide on how you can accomplish this task using the PnP Framework and C#.
What You’ll Learn
- What SharePoint Online is ?
- Understanding the PnP Framework
- Basics of C# for this task
- Step-by-step guide to check if a folder exists and create one if it doesn’t
What is SharePoint Online?
SharePoint Online is part of Microsoft 365, a cloud-based service that allows you to share and collaborate on documents, manage projects, and build corporate intranets. Think of it as a Swiss army knife for enterprise content management.
Understanding the PnP Framework
PnP (Patterns and Practices) is a collection of tools, guidance, and library provided by Microsoft that helps make it easier to work with SharePoint. By using the PnP Framework, you can streamline many tasks that would otherwise be complicated and time-consuming.
Basics of C# for SharePoint Online Tasks
C# is a versatile and widely-used programming language developed by Microsoft. It’s particularly strong in developing web applications and is extensively used for backend services and APIs. In the context of SharePoint Online, C# is utilized to integrate and manipulate SharePoint resources through code.
Step-by-Step Guide: How to Create Folders in Microsoft SharePoint using the PnP Framework and C#?
Prerequisites
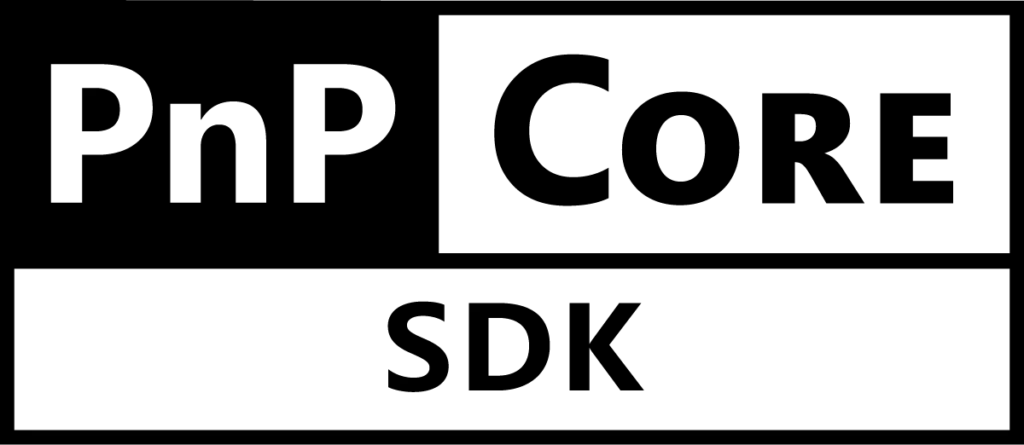
Before diving in, make sure you have the following:
- Microsoft 365 Account: Ensure you have access to SharePoint Online.
- Visual Studio Installed: We’ll be using Visual Studio for our C# coding.
- PnP Core SDK: This makes it easier to write your SharePoint Online operations.
Setting up the Environment
- Open Visual Studio: Start by creating a new Console App project.
- Add Dependencies: Add references to
PnP.Core.Auth
andPnP.Core.SDK
via NuGet Package Manager.
Install-Package PnP.Core.Auth
Install-Package PnP.Core.SDK
- Authentication: Set up authentication to access SharePoint.
using PnP.Framework;
using PnP.Framework.Authentication;
Provide credentials and set up your client context.
Code to Check and Create Folder
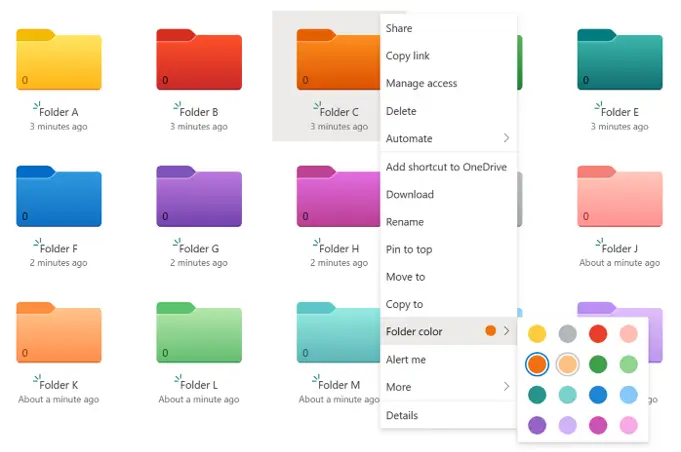
Now, let’s get to the code for PnP Framework Sharepoint Authentication c#:
using System;
using PnP.Core.Auth;
using PnP.Core.Model.SharePoint;
using PnP.Core.Services;
using PnP.Framework;
namespace CheckAndCreateFolder
{
class Program
{
static async Task Main(string[] args)
{
string siteUrl = \"https://yoursharepointsite.sharepoint.com\";
string folderName = \"MyFolder\";
var authProvider = new UsernamePasswordAuthenticationProvider(\"your-username\", \"your-password\");
using (var context = await PnPService.CreateAsync(new PnPContextOptions { SiteUrl = siteUrl, AuthenticationProvider = authProvider }))
{
var web = context.Web;
await web.LoadAsync(w => w.Lists);
var list = web.Lists.FirstOrDefault(l => l.Title == \"Documents\");
if (list != null)
{
var folder = await list.RootFolder.EnsureFolderAsync(folderName);
Console.WriteLine($\"Folder '{folderName}' {(folder != null ? \"exists or created successfully\" : \"could not be created\")}\");
}
else
{
Console.WriteLine(\"List 'Documents' not found.\");
}
}
}
}
}
Explanation
- Authentication: The
UsernamePasswordAuthenticationProvider
class handles authentication to your SharePoint Online site. - PnP Context Setup: Using the
PnPService.CreateAsync()
method initializes the context for SharePoint operations. - Folder Check and Creation: The
EnsureFolderAsync()
method checks if a folder exists under the given document library and creates it if it doesn’t.
Testing the Code
Execute the program, and verify the output:
- If the folder already exists, you’ll get a confirmation.
- If not, the program will create the folder and confirm its creation.
Common Issues and Troubleshooting
Authentication Errors
Ensure your credentials are correct and that you have the necessary permissions to access SharePoint.
List Not Found
Double-check the document library title. It should be exactly \”Documents\” or whatever you have named your default document library.
Package Issues
Ensure all necessary packages are correctly installed and up to date. Use the NuGet Package Manager to verify.
Conclusion
By following this guide, you should now be able to check for the existence of a folder in SharePoint Online and create one if it doesn’t exist. Utilizing the PnP Framework with C# can streamline numerous operations in SharePoint and make your tasks much simpler.
FAQs
Q1: Do I need to know advanced C# to follow this guide?
No, basic understanding of C# and programming concepts will suffice.
Q2: Can I use a different authentication method?
Yes, the PnP Framework supports various authentication methods, including OAuth and SharePoint App Authentication.
Q3: Is PnP Framework supported in SharePoint on-premises?
Yes, but with some limitations and variations in the setup process.
Q4: Can I use this method for other types of lists besides the Document Library?
Absolutely! Just adjust the code to target the specific list name you’re interested in.
Q5: What if I encounter a ‘Throttling’ issue?
Implement retry logic to handle throttling scenarios, especially for extensive loops or large datasets.
And there you have it! Happy coding and enjoy making the most out of SharePoint Online!
#MSFTAdvocate #AbhishekDhoriya #LearnWithAbhishekDhoriya #DynamixAcademy
References & Read More
- Upgrading your code from SharePoint Client Side Object Model (CSOM) to the PnP Libraries
- Discover the Database Version of Dataverse
- Unlocking SharePoint Online API with Azure AD App-Only Permissions: A Beginner’s Guide to Certificate Authentication
- Demystifying Dynamics 365 Business Central Integration with Microsoft Dataverse: Your Ultimate Beginner’s Guide
- Unlocking the Future: 2024 Release Wave 2 Plans for Microsoft Dynamics 365 and Microsoft Power Platform
2 thoughts on “Master How to Create Folders in SharePoint Online with PnP Framework and C#?”