How to Retrieve Multiple Records Using Web API in Dynamics 365 Version 9.0?
Hey there! If you’re looking to fetch multiple records from Dynamics 365 Online using JavaScript and WebAPI, you’ve come to the right place. Let’s break this down step by step to make it easy and straightforward.
Dynamics 365 Web API Overview: The Dynamics 365 Web API in Customer Engagement is a cool tool for developers to use across many programming languages and devices. It’s based on the OData protocol, which is like a set of rules for building and using APIs that work with lots of data.
Step 1: Setting Up Your Environment (Dynamics 365 Customer Engagement)
Before jumping into the code, ensure you have your Dynamics 365 environment ready and you have access to the JavaScript files where you’ll write your code. You also need the necessary permissions to retrieve data from the entities you’re working with.
Step 2: Understanding WebAPI and OData
Dynamics 365 WebAPI is a RESTful service that allows you to interact with data in Dynamics 365 using HTTP requests. OData (Open Data Protocol) is used to query and manipulate data, making it powerful and flexible for your data management needs.
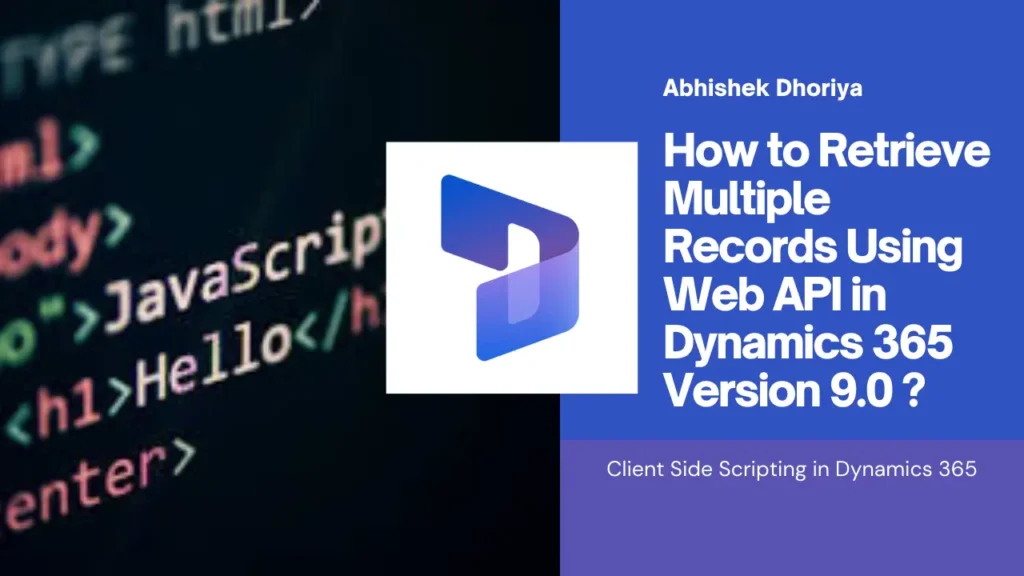
Step 3: Writing the JavaScript Code (Dynamics 365 Web API example)
Let’s get into writing the JavaScript function to retrieve multiple records. Here’s a basic example to get you started: GitHub Source : D365WebAPIRetreiveMultipleJS.js
var scripting = {
retrieveMultipleContacts(executioncontext) {
debugger;
var formContext = executioncontext.getFormContext();
var accountId = formContext.data.entity.getId();
var fetchXml =
"<fetch version='1.0' output-format='xml-platform' mapping='logical' distinct='false'><entity name='contact' ><attribute name='fullname' /><attribute name='telephone1' /><attribute name='contactid' /><order attribute='fullname' descending='false' /><filter type='and'><condition attribute='parentcustomerid' operator='eq' uitype='account' value ='" +
accountId +
"' /></filter></entity ></fetch > ";
Xrm.WebApi.retrieveMultipleRecords('contact', 'fetchXml= ' + fetchXml).then(
function success(result) {
for (var i = 0; i < result.entities.length; i++) {
console.log(result.entities[i]);
}
},
function (error) {
console.log(error.message);
}
);
},
};
Explanation of the Code:
- Creating XMLHttpRequest Object: We start by creating a new instance of
XMLHttpRequest
, which allows us to send HTTP requests to the server. - Configuring the Request: The
open
method initializes the request. We use the HTTP GET method to fetch data. The URL includes the Dynamics 365 base URL, the entity name, and the query to filter the records we want to retrieve. - Setting Request Headers: The headers specify parameters like OData version and content type to ensure the request is correctly formatted and understood by the Dynamics 365 server.
- Handling the Response: The
onreadystatechange
function is triggered when the state of the request changes. When the request is complete (readyState === 4
), we check the status. If it’s 200 (OK), we parse the JSON response and log the results. - Sending the Request: Finally, the
send
method sends the request to the server.
Step 4: Using the Function
You can call this function to retrieve data from any entity by passing the entity name and the query string. Here’s an example:
Xrm.WebApi.retrieveMultipleRecords(entityLogicalName,options,maxPageSize).then(successCallback, errorCallback);
Replace "accounts"
with the name of the entity you’re working with and query
with the actual OData query you need.
Step 5: Handling the Retrieved Data (Dynamics 365 Web API JavaScript example)
Once the records are retrieved, you can use the data as needed. You might want to display it on the UI, use it in further logic, or log it for debugging. Here’s how you might extend the function to handle the retrieved data:
var scripting = {
retrieveMultipleContacts(executioncontext) {
debugger;
var formContext = executioncontext.getFormContext();
var accountId = formContext.data.entity.getId();
var fetchXml =
"<fetch version='1.0' output-format='xml-platform' mapping='logical' distinct='false'><entity name='contact' ><attribute name='fullname' /><attribute name='telephone1' /><attribute name='contactid' /><order attribute='fullname' descending='false' /><filter type='and'><condition attribute='parentcustomerid' operator='eq' uitype='account' value ='" +
accountId +
"' /></filter></entity ></fetch > ";
Xrm.WebApi.retrieveMultipleRecords('contact', 'fetchXml= ' + fetchXml).then(
function success(result) {
for (var i = 0; i < result.entities.length; i++) {
console.log(result.entities[i]);
}
},
function (error) {
console.log(error.message);
}
);
},
};
In this example, the retrieved records are logged to the console, and each record’s name and account number are displayed.
Additional Tips and Tricks while using Dynamics 365 Web API
- Async/Await: For cleaner code, consider using
async
andawait
to handle asynchronous calls. - Error Handling: Always include comprehensive error handling to manage issues like network errors, invalid queries, or permissions problems.
- Pagination: If you need to retrieve a large number of records, consider implementing pagination using OData query options like
$skip
and$top
. - OData Details: You can learn more about OData, which is a standard way to create APIs, by visiting the OData website. It’s pretty neat because it lets you access and share data in a consistent way.
- Unified API for All: Whether you’re working with Customer Engagement (on-premises) or Dataverse, you get to use the same Web API. Isn’t that convenient? You can find all the documentation you need on the Power Apps website.
- Freedom to Choose: Since the Web API uses open standards, there aren’t any specific libraries provided. But don’t worry, you can find a bunch of third-party libraries that support OData and start creating awesome stuff in your favorite programming language
That’s it! Now you have a detailed guide on how to retrieve multiple records in Dynamics 365 Online using JavaScript and WebAPI. Give it a try and feel free to reach out if you have any questions. Happy coding!
What is the Web API in Dynamics 365?
The Web API in Dynamics 365 is a RESTful service that uses HTTP protocol and OData v4 standard. It allows developers to query a Dataverse table and integrate Dynamics 365 with other systems.
Where to find Dynamics 365 Web API URL?
To locate the Web API URL for your Dynamics 365 setup, follow these steps:
Open your Dynamics 365 instance.
Head over to Settings, then Customizations, and finally Developer Resources.
You’ll find the API URL in the Service Root URL section.
You can use this URL as required. For instance, to access the Web API via a browser, simply paste this URL into the browser’s address bar.
The URL generally follows this format: https://yourorg.crm.dynamics.com/api/data/v9.0/
, where “yourorg” should be replaced with your organization’s name.
Does Dynamics 365 have REST API?
Dynamics 365 does indeed have REST APIs. Think of these as a set of tools that let you interact with Dynamics 365, just like you’d chat with a friend. You can use them to get data, change data, and do all sorts of cool stuff with your Dynamics 365 apps. They’re pretty easy to use, work with many programming languages, and are a great way to make Dynamics 365 work just the way you want it to. So yes, they’re pretty awesome!
How to call REST API in Dynamics 365?
To use a REST API in Dynamics 365, you first prepare your request with the right URL and headers. Then, you send it off using a method like GET or POST. When you get a response back, you can use the data however you need. It’s like sending a letter and waiting for a reply. And don’t worry, there are lots of guides and examples to help you out. Happy coding!
7 thoughts on “How to Retrieve Multiple Records Using Web API in Dynamics 365 Version 9.0?”